Dive Board
-
Python coding
Python coding
by Randyarvelo on Aug 17th, 2023 19:37 PM
[color=#374151][size=3][font=Söhne, ui-sans-serif, system-ui, -apple-system, "Segoe UI", Roboto, Ubuntu, Cantarell, "Noto Sans", sans-serif, "Helvetica Neue", Arial, "Apple Color Emoji", "Segoe UI Emoji", "Segoe UI Symbol", "Noto Color Emoji"]Can anyone provide recommendations for efficient strategies to optimize runtime performance in Python coding? Specifically, I'm looking for insights on techniques, libraries, or best practices that can help enhance the speed and efficiency of my Python programs. Any advice on improving code execution speed would be greatly appreciated![/font][/size][/color]
Randyarvelo
Posts: 3
Joined: 17.08.2023
Re: Python coding
by saith2562 on Aug 24th, 2023 13:50 PM
[color=#374151][size=3][font=Söhne, ui-sans-serif, system-ui, -apple-system, "Segoe UI", Roboto, Ubuntu, Cantarell, "Noto Sans", sans-serif, "Helvetica Neue", Arial, "Apple Color Emoji", "Segoe UI Emoji", "Segoe UI Symbol", "Noto Color Emoji"]Absolutely, there are several strategies and techniques you can employ to optimize the runtime performance of your Python code. Here's a compilation of best practices and tools to help enhance the speed and efficiency of your programs:[/font][/size][/color]
[color=#374151][size=3][font=Söhne, ui-sans-serif, system-ui, -apple-system, "Segoe UI", Roboto, Ubuntu, Cantarell, "Noto Sans", sans-serif, "Helvetica Neue", Arial, "Apple Color Emoji", "Segoe UI Emoji", "Segoe UI Symbol", "Noto Color Emoji"][color=#000000][size=2][font=docs-Roboto]mypascoconnect[/font][/size][/color][/font][/size][/color]
[ol]
[li][left][color=var(--tw-prose-bold)]Use Efficient Data Structures:[/color]
[ul]
[li]Choose the appropriate data structures for your tasks. Lists, dictionaries, sets, and arrays have different time complexities for various operations.[/li]
[li]Use sets for membership tests, dictionaries for fast lookups, and lists for sequential data.[/li]
[/ul]
[/left]
[/li]
[li][left][color=var(--tw-prose-bold)]Algorithm Complexity:[/color]
[ul]
[li]Opt for algorithms with lower time complexities (e.g., O(n log n), O(n), etc.) for tasks like sorting and searching.[/li]
[li]Avoid nested loops whenever possible, as they can lead to exponential time complexity.[/li]
[/ul]
[/left]
[/li]
[li][left][color=var(--tw-prose-bold)]NumPy and pandas:[/color]
[ul]
[li]If you're working with large datasets or numerical computations, use the NumPy and pandas libraries. They provide optimized data structures and operations.[/li]
[/ul]
[/left]
[/li]
[li][left][color=var(--tw-prose-bold)]List Comprehensions and Generators:[/color]
[ul]
[li]Utilize list comprehensions for creating lists efficiently and generators for lazy evaluation. These can save memory and time compared to traditional loops.[/li]
[/ul]
[/left]
[/li]
[li][left][color=var(--tw-prose-bold)]Cython and Numba:[/color]
[ul]
[li]Cython and Numba are tools that can speed up your Python code by compiling it to C or optimizing it for performance.[/li]
[/ul]
[/left]
[/li]
[li][left][color=var(--tw-prose-bold)]Profiling and Optimization Tools:[/color]
[ul]
[li]Use profiling tools likecProfile
andline_profiler
to identify bottlenecks in your code.[/li]
[li]Optimization tools likePyPy
andCython
can help speed up certain parts of your codebase.[/li]
[/ul]
[/left]
[/li]
[li][left][color=var(--tw-prose-bold)]Vectorization:[/color]
[ul]
[li]Whenever possible, use vectorized operations instead of explicit loops. Libraries like NumPy excel at this.[/li]
[/ul]
[/left]
[/li]
[li][left][color=var(--tw-prose-bold)]Concurrency and Parallelism:[/color]
[ul]
[li]Python'sconcurrent.futures
andmultiprocessing
modules allow you to run tasks concurrently or in parallel, utilizing multiple CPU cores.[/li]
[/ul]
[/left]
[/li]
[li][left][color=var(--tw-prose-bold)]Cache Results:[/color]
[ul]
[li]If parts of your code involve expensive calculations, consider caching the results so that you don't have to recompute them.[/li]
[/ul]
[/left]
[/li]
[li][left][color=var(--tw-prose-bold)]Avoid Global Variables:[/color]
[ul]
[li]Accessing global variables can be slower than accessing local variables. Minimize their use when possible.[/li]
[/ul]
[/left]
[/li]
[li][left][color=var(--tw-prose-bold)]Use Built-in Functions:[/color]
[ul]
[li]Python's built-in functions and libraries are usually optimized for performance. Utilize them instead of re-inventing the wheel.[/li]
[/ul]
[/left]
[/li]
[li][left][color=var(--tw-prose-bold)]Memory Management:[/color]
[ul]
[li]Be mindful of memory usage. Large objects can slow down your code due to memory swapping.[/li]
[/ul]
[/left]
[/li]
[li][left][color=var(--tw-prose-bold)]Optimize Loops:[/color]
[ul]
[li]Move invariant computations outside the loop.[/li]
[li]Preallocate lists when their size is known in advance.[/li]
[/ul]
[/left]
[/li]
[li][left][color=var(--tw-prose-bold)]Lazy Evaluation:[/color]
[ul]
[li]Use lazy evaluation when processing large data sets, which can help avoid unnecessary calculations.[/li]
[/ul]
[/left]
[/li]
[li][left][color=var(--tw-prose-bold)]Regular Expressions:[/color]
[ul]
[li]While powerful, regular expressions can be slow for complex patterns. Consider using alternative string methods for simpler tasks.[/li]
[/ul]
[/left]
[/li]
[li][left][color=var(--tw-prose-bold)]Avoid Function Calls in Loops:[/color]
[ul]
[li]Minimize the number of function calls within loops, as they can introduce overhead.[/li]
[/ul]
[/left]
[/li]
[li][left][color=var(--tw-prose-bold)]Keep External Calls Minimal:[/color]
[ul]
[li]External calls like file I/O and API requests can be slow. Minimize these when possible.[/li]
[/ul]
[/left]
[/li]
[li][left][color=var(--tw-prose-bold)]Choose the Right Libraries:[/color]
[ul]
[li]Research and choose libraries that are well-optimized for your specific use case.[/li]
[/ul]
[/left]
[/li]
[/ol]
[color=#374151][size=3][font=Söhne, ui-sans-serif, system-ui, -apple-system, "Segoe UI", Roboto, Ubuntu, Cantarell, "Noto Sans", sans-serif, "Helvetica Neue", Arial, "Apple Color Emoji", "Segoe UI Emoji", "Segoe UI Symbol", "Noto Color Emoji"]Remember, optimization should be guided by profiling. Profile your code to identify bottlenecks before making optimizations, as it helps you focus on the parts that truly need improvement. Also, keep in mind that premature optimization might lead to less readable code, so always prioritize code clarity and maintainability.[/font][/size][/color]saith2562
Posts: 3
Joined: 17.08.2023
STATISTICS
Total posts: 188442
Total topics: 45177
Total members: 46687
Newest member: Daniel G.
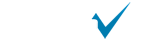
Rencontrez la famille Durovis Dive et transformer votre appareil mobile en un casque de réalité virtuelle. jeux d'expérience et 360 vidéos ° en VR sur votre propre smartphone ou tablette.
Modes de paiement acceptés

